<script src="zepto.js"></script>
<script src="jhash.js"></script>
<script src="fastClick.js"></script>
<script src="iScroll.js"></script>
<script src="underscore.js"></script>
<script src="handlebar.js"></script>
<script src="datacenter.js"></script>
<script src="util/wxbridge.js"></script>
<script src="util/login.js"></script>
<script src="util/base.js"></script>
于是后jQuery时代,国内流行三种模块机制,以seajs主体的CMD,以requirejs为主体的AMD,及nodejs自带的Commonjs。当然,后来还有一种三合一方案UMD(AMD, Commonjs与es6 modules)。
requirejs的定义与使用:
define(['jquery'], function($){
//some code
var mod = require("./relative/name");
return {
//some code
} //返回值可以是对象、函数等
})
require(['cores/cores1', 'cores/cores2', 'utils/utils1', 'utils/utils2'], function(cores1, cores2, utils1, utils2){
//some code
})
requirejs是世界第一款通用的模块加载器,尤其自创了shim机制,让许多不模范的JS文件也可以纳入其加载系统。
define(function(require){
var $ = require("jquery");
$("#container").html("hello,seajs");
var service = require("./service")
var s = new service();
s.hello();
});
//另一个独立的文件service.js
define(function(require,exports,module){
function Service(){
console.log("this is service module");
}
Service.prototype.hello = function(){
console.log("this is hello service");
return this;
}
module.exports = Service;
});
Seajs是阿里大牛玉伯加的加载器,借鉴了Requiejs的许多功能,听说其性能与严谨性超过前者。当前为了正确分析出define回调里面的require语句,还发起了一个 100 美刀赏金活动,让国内高手一展身手。
https://github.com/seajs/seajs/issues/478
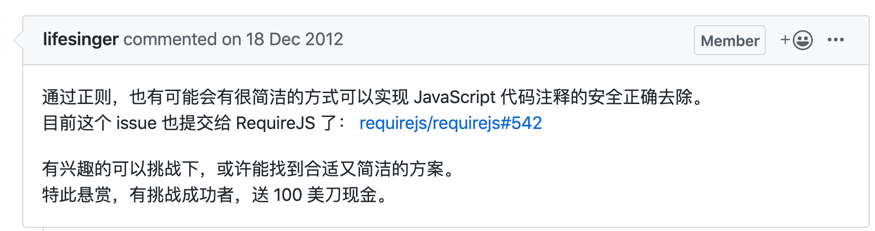
相对而言,nodejs模块系统就简单多了,它没有专门用于包裹用户代码的define方法,它不需要显式声明依赖。
//world.js
exports.world = function() {
console.log('Hello World');
}
//main.js
let world = require('./world.js')
world();
function Hello() {
var name;
this.setName = function(thyName) {
name = thyName;
};
this.sayHello = function() {
console.log('Hello ' + name);
};
};
module.exports = Hello;
而官方钦点的es6 modules与nodejs模块系统极其相似,只是将其方法与对象变成关键字。
//test.js或test.mjs
import * as test from './test';
//aaa.js或aaa.mjs
import {aaa} from "./aaa"
const arr = [1, 2, 3, 4];
const obj = {
a: 0,
b: function() {}
}
export const foo = () => {
const a = 0;
const b = 20;
return a + b;
}
export default {
num,
arr,
obj,
foo
}
那怎么使用呢?根据规范,浏览器需要在link标签与script标签添加新的属性或属性值来支持这新特性。(详见:https://www.jianshu.com/p/f7db50cf956f)
<link rel="modulepreload" href="lib.mjs">
<link rel="modulepreload" href="main.mjs">
<script type="module" src="main.mjs"></script>
<script nomodule src="fallback.js"></script>
但可惜的是,浏览器对模块系统的支持是非常滞后,并且即便最新的浏览器支持了,我们还是免不了要兼容旧的浏览器。对此,我们只能奠出webpack这利器,它是前端工程化的集大成者,可以将我们的代码通过各种loader/plugin打包成主流浏览器都认识的JavaScript语法,并以最原始的方式挂载进去。